Imagine being able to bring your ideas to life, automate tasks, and solve complex problems—all with just a few lines of code. Python makes it possible.
And the best part? You don’t need to be an expert to get started.
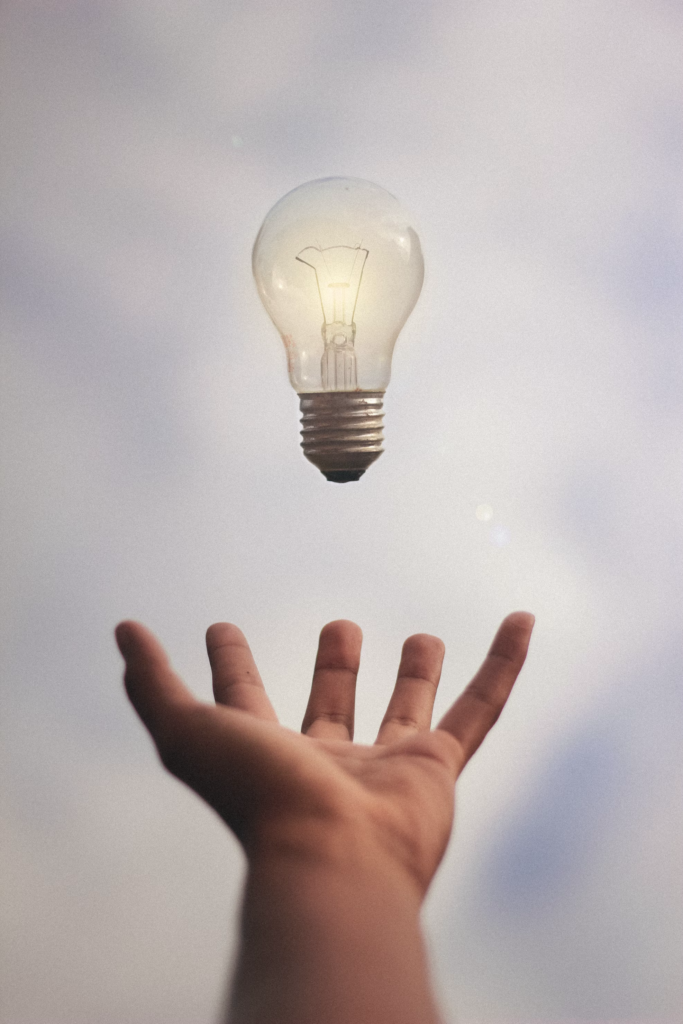
Python is a popular programming language for a good reason. It’s as close to the English language as you can get.
What’s beautiful about a high-level language like Python is that it handles the complex, behind-the-scenes details—like memory management and tricky syntax—so you can focus on expressing your ideas in a way that’s easy to understand.
Instead of worrying about how the computer processes your instructions, Python lets you focus on what you want to achieve. It makes the process feel less like you’re wrestling with complex code and more like you’re solving real-world problems.
But programming can be intimidating.
I remember feeling hesitant and overwhelmed when I first considered diving into coding.
Imposter syndrome was real. I didn’t think I was smart enough to learn a programming language.
However, once I pulled back the curtain, I realized it wasn’t as daunting as I’d imagined.
I started breaking problems into smaller, more manageable chunks, and before I knew it, I was fascinated by what I could accomplish.
I think the hardest part is just getting started. Once you begin, you’ll likely develop your own curiosity and excitement about what you can create with code.
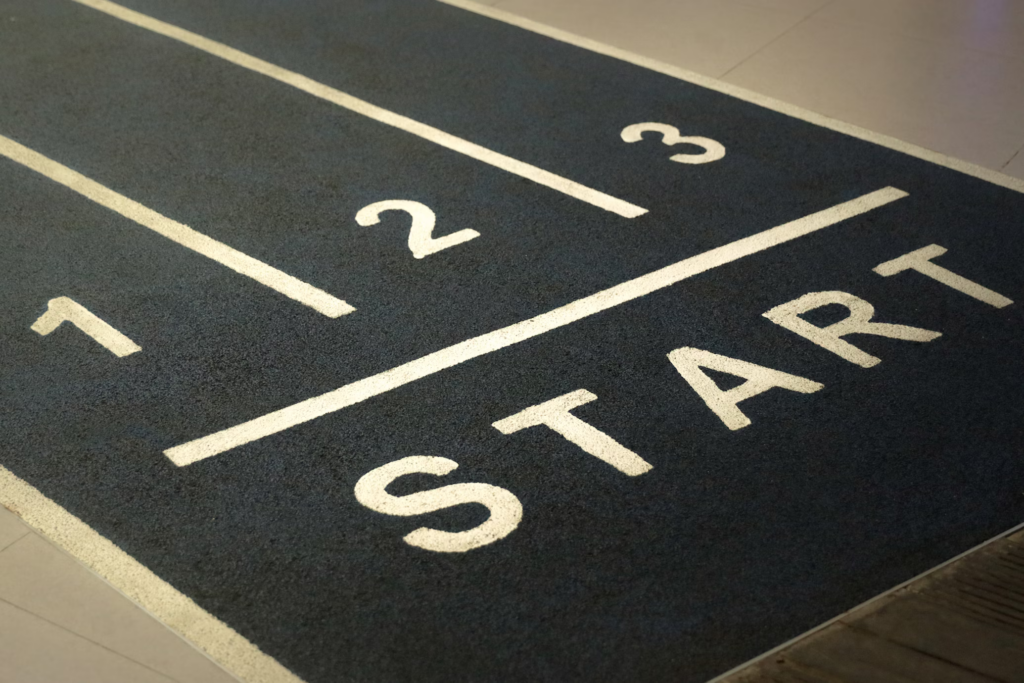
Code is powerful.
If you can dream it, you can build it.
And I’ll show you how you can get started!
The Ultimate Free Python Book for Beginners
That’s right, you read that correctly. I’m about to give you a free Python book that will be your guide in your programming journey.
I always recommend to buy a book if you love it enough, but here’s the free version: Python Crash Course.
And here’s the same book on Amazon below. You’ll notice this is the 3rd edition which is the latest at the time of this writing. The free version is the 2nd edition, but the differences are pretty small.
This book is wonderful because I found it has the most clear explanations of fundamental concepts in Python.
What’s also great is it gives you projects to work on that are fun, educational, and very informative.
Starting from the very basics of Python, like variables, loops, and data structures, and builds up to more advanced topics. This structured progression makes it incredibly easy to follow, even if you’ve never written a line of code before.
Eric Matthes does an excellent job of breaking down complex concepts into simple, easy-to-understand explanations. His writing is clear, engaging, and free of jargon, which makes it perfect for beginners who might feel overwhelmed by technical language.
Throughout the book, Matthes uses real-world examples that are simple and practical. You’ll learn Python through examples that help you solve real problems, from writing programs to automating simple tasks to building small projects. This practical approach makes Python feel useful and relevant right away.
Matthes encourages good programming habits from the very beginning, teaching you how to write clean, readable, and efficient code. You’ll learn about debugging techniques, using version control (Git), and writing tests, which are essential skills as you advance in your programming career.
“Python Crash Course” assumes no prior programming experience, making it ideal for beginners. Whether you’ve never coded before or you’re just switching to Python, you can start from scratch and quickly build up your skills without feeling lost.
Why Jupyter Notebooks Are Perfect for Learning Python: Interactive, Visual, and Easy to Use
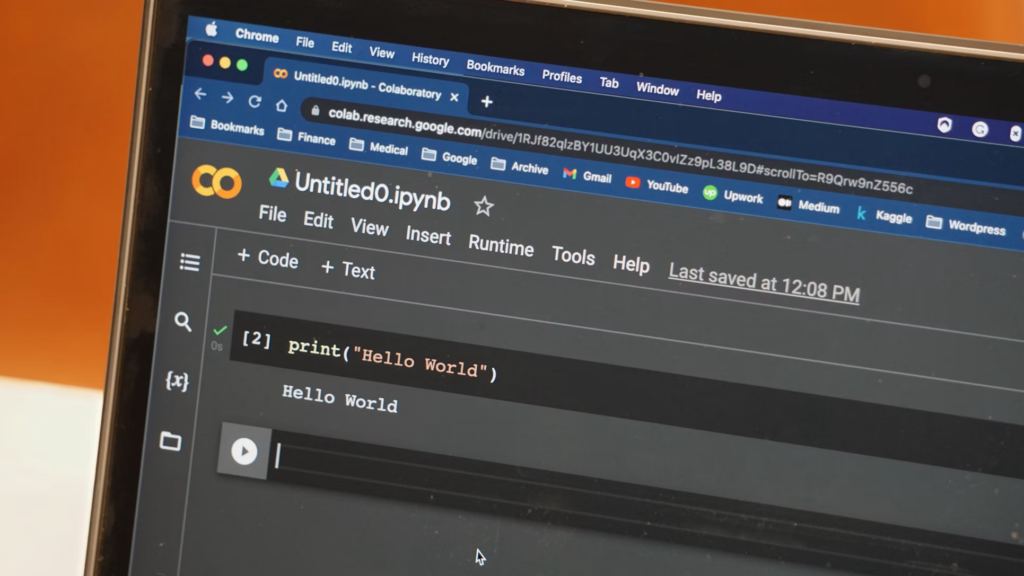
A Jupyter Notebook is an interactive, typically web-based environment that allows you to write and run Python code in small, manageable sections (called cells).
Google Colab (short for “Collaboratory”) is a cloud-based platform that allows you to write and run Python code in a notebook-style format. It’s also free!
It’s like a Google Docs or a Word document, but for code. You can write, execute, and share Python code, along with text and images, all in the same document.
You don’t need to worry about setting up your environment, as you would typically have to do on your local computer. Additionally, since Colab runs in the cloud, you’re not limited by your computer’s resources. This is especially beneficial for running heavy computations, as you can take advantage of free access to GPUs/TPUs.
To get started, open a new Google Colab notebook.
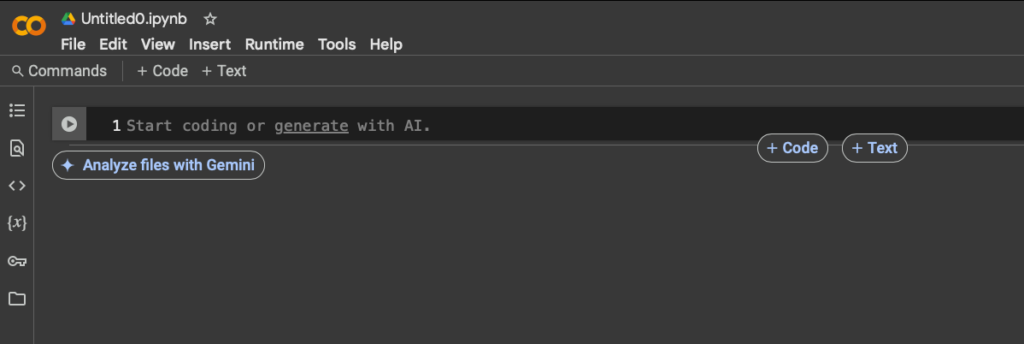
On the top left, you’ll notice the default title name of the file and the extension (.ipynb). That file extension means it’s a Jupyter notebook. You can change the title here if you want now or later.
In the first cell you can see it’s empty and you’re prompted to start coding.
You can enter Python code directly in this cell and click the play button on the left to see the output. Or you can add a new cell by clicking the +Code
button.
You can also add a new text cell by clicking the +Text
button.
A text cell contains markdown code. Markdown is a lightweight markup language that is used to format plain text, making it easy to create formatted documents
When you’re writing a document or taking notes, you often want to organize the information into different levels, like chapters, sections, and subsections. Using headings helps you do that.
Think of headings like a “table of contents” for your notebook or document. The more important topics get larger titles, while smaller, more specific topics get smaller titles. Here’s how it works:
- Main Topic: The most important heading, like the title of a chapter, is the biggest (Heading 1). This is for your main topic.
# Heading 1
→ Main topic
- Subtopics: If you have a smaller topic under the main one, you use a smaller heading (Heading 2). It’s like breaking up the main chapter into sections.
## Heading 2
→ Subtopic under Heading 1
- Details under Subtopics: If you need even more details under a subtopic, you keep going smaller with the headings (Heading 3, Heading 4, etc.).
### Heading 3
→ Details or examples under Heading 2#### Heading 4
→ More specific details under Heading 3
Each time you add one more #
, the heading gets smaller and shows that it’s a more detailed or specific point within the bigger topic.
That’s all you need to get started. You can begin coding in Python and not worry about setting up the environment!
Advanced Usage
To truly appreciate the power of Python, it’s best to see it in action with a practical example. Since you’re already in the Google ecosystem, you can easily interact with files in your Google Drive directly within your notebook!
On the left hand side, you’ll see menu options. One of them is a folder icon, click on it.
Google should automatically mount your Google drive here. If not, you can simply click the mount drive button at the top once you’ve clicked the folder icon.
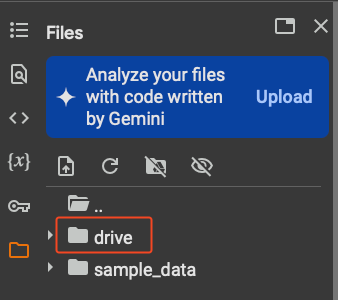
Your drive folder will simply be called “drive”.
Here you have access to any files and you can interact with these files using any packages in Python.
For example, if I want to learn the Pandas package and convert a Google sheets file to a Pandas DataFrame, then I can read in that file path using pd.read_excel()
method.
But first let’s get the path to your file.
import os
# First let's get the contents of your drive by creating a path to your drive
drive_path = "/content/drive/MyDrive/"
# List the contents in the drive
files_and_folders = os.listdir(drive_path)
print(files_and_folders)
If you enter the above code into a cell and run it, you should see the contents of your Google drive (i.e., files and folders).
If your file is in a folder, let’s navigate inside:
# Create a path to the folder
target_folder = os.path.join(drive_path, "Your Folder Name")
# Let's look at the files inside this folder
target_folder_files = os.listdir(target_folder)
print(target_folder_files)
This will show you all the files in your folder. Alternatively, if your Excel file is already at the root of your Google drive directory then you can just get the path there:
# Directly get the file path
file_path = os.path.join(drive_path, "your_google_sheet_file.xlsx")
Next, let’s read in that Excel file into a Pandas DataFrame:
# Create a string path to the file you want to read as a DataFrame inside a folder
file_path_in_folder = os.path.join(target_folder, "your_file_inside_folder.xlsx")
# Read in an excel file as a Pandas DataFrame object
# Or use the file_path variable that's already in the root of your Google drive
df = pd.read_excel(file_path_in_folder)
# Display the DataFrame
display(df)
You should see a table appear below in your cell with the data within that file. Congrats, you’re now ready to slice and dice your data using Pandas!
I highly recommend to have the Python Crash Course open in another window and code along the book.
Real-Time Collaboration with Notebooks
The only downside to Google colab notebooks is that real-time collaboration is pretty much non-existent.
You can share a Google colab notebook with a colleague or friend and both of you can edit it in real-time. But the changes have significant lag that defeats the whole purpose of working with someone.
Which is why I recommend a great alternative called Deepnote.
It’s heavily geared towards Data Scientists, but nobody is gate keeping here. Use the tools you need to learn Python!
Their free tier is sufficient for most needs and the best part is you can code with a friend in real-time using their notebooks.
I know it’s probably annoying to have to switch platforms, but it can be worth it if you’re trying to learn from a programming buddy or mentor.
I recommend not using their AI since it wouldn’t help your learning. If possible I would turn that setting off or just ignore it.
Wrapping Up: Key Takeaways and Next Steps
Learning Python has never been easier, thanks to the technology we have today.
There’s an abundance of documentation, YouTube tutorials, and free resources available to help you learn programming.
Python is a lot of fun. Its simple syntax and ease of use make it incredibly powerful when you know how to wield it.
It’s like unlocking a superpower for your daily tasks. Once you learn how to program, you’ll never look at a manual task the same way again — you’ll start automating everything!
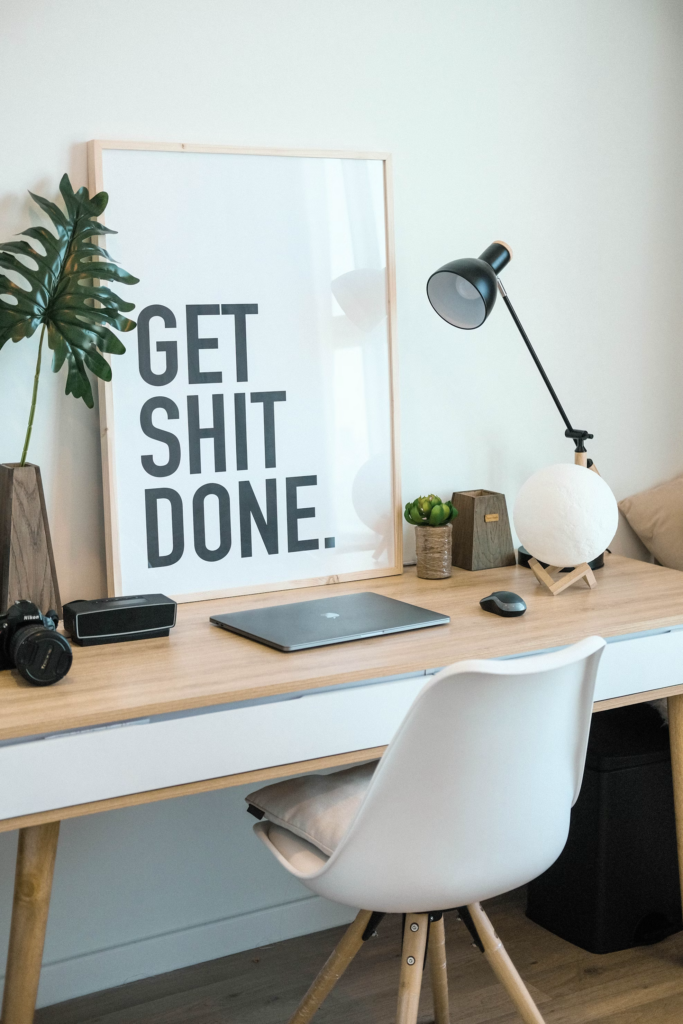
No matter what field you’re in, Python is a valuable skill to have in your toolkit. Everyone interacts with technology to some degree, and even if you’re not a tech expert, I guarantee you’ll find countless ways to use Python to your advantage.
You could even create your own personal assistant or bot to handle tasks for you, all for free.
Programming is a powerful tool and you don’t need to be a genius to learn it. You just need patience, the right resources, and a willingness to learn on your own.
Empower yourself by investing the time to learn, just like you would with any other skill you want to master.
Think of one task you do repeatedly and start brainstorming how you could automate it with code.
Whether it be for work, hobbies, or anything else, you can get creative and build a script to automate or semi-automate most processes that don’t involve too much oversight.
Start small, experiment, and see how far you can go. The more you learn, the more you’ll unlock your potential. So, what are you waiting for? Find your first task, and let’s get coding!
Leave a Reply